Browse Source
added a 'Player' class and a 'Game' class to achieve higher encapsulation and data abstraction
master
added a 'Player' class and a 'Game' class to achieve higher encapsulation and data abstraction
master
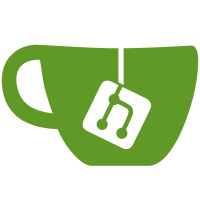
11 changed files with 206 additions and 25 deletions
-
3include/Controller.h
-
30include/Game.h
-
2include/Graphics.h
-
24include/Player.h
-
26src/Controller.cpp
-
75src/Game.cpp
-
6src/Graphics.cpp
-
43src/Player.cpp
-
8src/PointClassImpl.cpp
-
1src/SnakeImpl.cpp
-
13src/main.cpp
@ -0,0 +1,30 @@ |
|||||
|
#pragma once |
||||
|
|
||||
|
//This class will be the abstract Game Interface |
||||
|
#include <string> |
||||
|
#include "Controller.h" |
||||
|
#include "Snake.h" |
||||
|
#include "Player.h" |
||||
|
class SnakeGame{ |
||||
|
private: |
||||
|
std::string gameName; |
||||
|
std::list<Player> players; |
||||
|
unsigned int highScore; |
||||
|
std::string bestPlayer; |
||||
|
|
||||
|
void play(std::string playerName); |
||||
|
void addPlayer(std::string playerName); |
||||
|
|
||||
|
public: |
||||
|
SnakeGame(); //intialize graphics and set the game screen |
||||
|
~SnakeGame(); |
||||
|
|
||||
|
|
||||
|
unsigned int getHighScore(void); |
||||
|
std::string getBestPlayer(void); |
||||
|
|
||||
|
//print the statistics (highest score & games played) of each player |
||||
|
//void printGameStatistics(void); |
||||
|
|
||||
|
void play(void); |
||||
|
}; |
@ -0,0 +1,24 @@ |
|||||
|
#pragma once |
||||
|
|
||||
|
#include <iostream> |
||||
|
#include <string> |
||||
|
#include "Controller.h" |
||||
|
|
||||
|
class Player{ |
||||
|
private: |
||||
|
std::string name; |
||||
|
unsigned int points; |
||||
|
unsigned int timesPlayed; |
||||
|
public: |
||||
|
Player(); |
||||
|
Player(std::string _name); |
||||
|
~Player(); |
||||
|
|
||||
|
std::pair<std::string, unsigned int> play(void); |
||||
|
|
||||
|
//Point managment methods |
||||
|
unsigned int getPoints(void); |
||||
|
void addPoints(unsigned int _points); |
||||
|
|
||||
|
std::string getName(void); |
||||
|
}; |
@ -0,0 +1,75 @@ |
|||||
|
#include "Game.h"
|
||||
|
#include <algorithm>
|
||||
|
using namespace std; |
||||
|
|
||||
|
SnakeGame::SnakeGame() |
||||
|
:gameName{"~Snake Game by VissaM~"},highScore{0}, bestPlayer{"None"} |
||||
|
{} |
||||
|
|
||||
|
SnakeGame::~SnakeGame(){} |
||||
|
|
||||
|
void SnakeGame::addPlayer(string playerName){ |
||||
|
players.emplace_back( Player{playerName} ); |
||||
|
} |
||||
|
|
||||
|
unsigned int SnakeGame::getHighScore(void){ |
||||
|
return highScore; |
||||
|
} |
||||
|
|
||||
|
string SnakeGame::getBestPlayer(void){ |
||||
|
return bestPlayer; |
||||
|
} |
||||
|
|
||||
|
void SnakeGame::play(string playerName){ |
||||
|
pair<string, unsigned int> curBest{playerName, 0}; |
||||
|
for(auto player : players){ |
||||
|
if(player.getName() == playerName){ |
||||
|
initializeGraphics((char *)gameName.c_str()); |
||||
|
curBest = player.play(); |
||||
|
endGraphics(); |
||||
|
break; |
||||
|
} |
||||
|
} |
||||
|
|
||||
|
if(curBest.second > highScore){ |
||||
|
highScore = curBest.second; |
||||
|
bestPlayer = curBest.first; |
||||
|
} |
||||
|
|
||||
|
cout << "Highscore: " << highScore << " by " << bestPlayer << "\n" <<endl; |
||||
|
} |
||||
|
|
||||
|
void SnakeGame::play(void){ |
||||
|
while(1){ |
||||
|
string playerName; |
||||
|
cout << "Who's playing: "; |
||||
|
cin >> playerName; |
||||
|
cout << endl; |
||||
|
|
||||
|
list<Player>::iterator p; |
||||
|
for(p=players.begin(); p!=players.end(); p++){ |
||||
|
if(p->getName() == playerName) |
||||
|
break; |
||||
|
} |
||||
|
|
||||
|
if(p == players.end()){ |
||||
|
//if the player isn't in the list, add him/her
|
||||
|
addPlayer(playerName); |
||||
|
} |
||||
|
|
||||
|
|
||||
|
play(playerName); //get the player to play the game
|
||||
|
|
||||
|
|
||||
|
cout << "Do you want to play again? (yes or no): "; |
||||
|
string ans; |
||||
|
|
||||
|
cin >> ans; |
||||
|
|
||||
|
if(ans != "yes"){ |
||||
|
cout << "Exiting ..." << endl; |
||||
|
break; |
||||
|
} |
||||
|
cout << "Perfect...\n" << endl; |
||||
|
} |
||||
|
} |
@ -0,0 +1,43 @@ |
|||||
|
#include "Player.h"
|
||||
|
|
||||
|
using namespace std; |
||||
|
|
||||
|
Player::Player() |
||||
|
: name{"Unknown"}, points{0}, timesPlayed{0} |
||||
|
{} |
||||
|
|
||||
|
Player::Player(string _name) |
||||
|
: name{_name}, points{0}, timesPlayed{0} |
||||
|
{} |
||||
|
|
||||
|
Player::~Player() |
||||
|
{/* No need to do something */} |
||||
|
|
||||
|
string Player::getName(void){ |
||||
|
return this->name; |
||||
|
} |
||||
|
|
||||
|
void Player::addPoints(unsigned int _points){ |
||||
|
points += _points; |
||||
|
} |
||||
|
|
||||
|
unsigned int Player::getPoints(void){ |
||||
|
return this->points; |
||||
|
} |
||||
|
|
||||
|
std::pair<std::string, unsigned int> Player::play(void){ |
||||
|
//here we will implement the basic loop of the game since each player has its remote controller
|
||||
|
//and can play the game
|
||||
|
Controller controller; |
||||
|
while (controller.wantsToQuit() == false) |
||||
|
{ |
||||
|
controller.readInput(); |
||||
|
if (controller.act() == DEFEAT) |
||||
|
break; |
||||
|
} |
||||
|
|
||||
|
points = controller.getCurrScore(); |
||||
|
controller.resetScore(); |
||||
|
|
||||
|
return {this->name, points}; |
||||
|
} |
@ -1,19 +1,12 @@ |
|||||
|
|
||||
#include <ncurses.h>
|
#include <ncurses.h>
|
||||
#include <iostream>
|
#include <iostream>
|
||||
#include "Controller.h"
|
|
||||
|
#include "Game.h"
|
||||
using namespace std; |
using namespace std; |
||||
|
|
||||
int main() { |
int main() { |
||||
initializeGraphics(); |
|
||||
Controller c; |
|
||||
/* CODE TO BE WRITTEN... */ |
|
||||
while(c.wantsToQuit() == false){ |
|
||||
c.readInput(); |
|
||||
if(c.act() == DEFEAT) |
|
||||
break; |
|
||||
} |
|
||||
endGraphics(); |
|
||||
|
SnakeGame game; |
||||
|
game.play(); |
||||
return 0; |
return 0; |
||||
|
|
||||
} |
} |
Write
Preview
Loading…
Cancel
Save
Reference in new issue