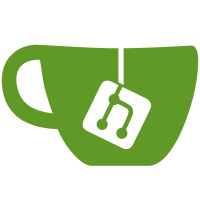
commit
d8018f647f
13 changed files with 736 additions and 0 deletions
-
24.vimspector.json
-
12CMakeLists.txt
-
41ReadMe.txt
-
50src/header-files/InputManager.h
-
26src/header-files/StorageEditor.h
-
60src/header-files/data-classes/Ware.h
-
47src/header-files/data-classes/WarenStorage.h
-
7src/header-files/pch.h
-
14src/main.cpp
-
264src/source-files/InputManager.cpp
-
30src/source-files/StorageEditor.cpp
-
69src/source-files/Ware.cpp
-
92src/source-files/WarenStorage.cpp
@ -0,0 +1,24 @@ |
|||
{ |
|||
"configurations": { |
|||
"Launch": { |
|||
"adapter": "vscode-cpptools", |
|||
"filetypes": [ "cpp", "c", "objc", "rust" ], // optional |
|||
"configuration": { |
|||
"request": "launch", |
|||
"program": "/home/leo/Documents/Code/kaufland/kaufland", |
|||
"cwd": "/home/leo/Documents/Code/kaufland", |
|||
"externalConsole": true, |
|||
"MIMode": "gdb" |
|||
} |
|||
}, |
|||
"Attach": { |
|||
"adapter": "vscode-cpptools", |
|||
"filetypes": [ "cpp", "c", "objc", "rust" ], // optional |
|||
"configuration": { |
|||
"request": "attach", |
|||
"program": "/home/leo/Documents/Code/kaufland/kaufland", |
|||
"MIMode": "gdb" |
|||
} |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,12 @@ |
|||
project(kaufland) |
|||
set (CMAKE_CXX_STANDARD 14) |
|||
|
|||
set(SOURCES |
|||
src/source-files/Ware.cpp |
|||
src/source-files/WarenStorage.cpp |
|||
src/source-files/StorageEditor.cpp |
|||
src/source-files/InputManager.cpp |
|||
) |
|||
|
|||
set(COMBINED_SOURCES ${SOURCES} src/main.cpp) |
|||
add_executable(kaufland ${COMBINED_SOURCES}) |
@ -0,0 +1,41 @@ |
|||
#Task: |
|||
|
|||
Ein Warenhaus der Marke Kaufland möchte ihr Warenmanagement von Papier auf Digital aufrüsten. |
|||
|
|||
- Sie haben 3 interne Warensegmente, eins für kühlung, eins für sperrige artikel und eins für Standardmaße (unter 20x20x20cm), |
|||
wobei nur das Warenhaus für sperrige Artikel solche Lagern kann die die standardmaße überschreiten |
|||
|
|||
- das Kühllager kann 10000, das Sperrlager 3500 und das Standardlager 25000 Produkte fassen |
|||
|
|||
- die Managementsoftware soll das eintragen, austragen und auflisten des aktuellen Warenhausstands ermöglichen |
|||
|
|||
- beim eintragen oder "Ware annehmen" wird ein Typ Produkt geliefert, bekannt sind dabei der Name, Produktnummer, Anzahl, Maße und Kühlungsbedarf |
|||
|
|||
- zwei LKW können auch das selbe Produkt liefern, wobei dann die Anzahl des Produkts erhöht werden muss anstatt es ein zweites mal ins system zu laden |
|||
|
|||
- das Warenhaus darf natürlich nicht über sein limit beladen werden |
|||
|
|||
- beim austragen wird eine Produktnummer und die Anzahl gegeben |
|||
|
|||
- beim auflisten wird, sortiert nach Warenhaustyp, die gesammten produkte mit ihren eigenschaften angegeben |
|||
|
|||
|
|||
#Project Structure: |
|||
|
|||
''' |
|||
src |
|||
├── header-files |
|||
│ ├── data-classes |
|||
│ │ ├── Ware.h |
|||
│ │ └── WarenStorage.h |
|||
│ ├── InputManager.h |
|||
│ ├── pch.h |
|||
│ └── StorageEditor.h |
|||
├── main.cpp |
|||
└── source-files |
|||
├── InputManager.cpp |
|||
├── StorageEditor.cpp |
|||
├── Ware.cpp |
|||
└── WarenStorage.cpp |
|||
|
|||
''' |
@ -0,0 +1,50 @@ |
|||
#include "pch.h" |
|||
#include "StorageEditor.h" |
|||
|
|||
class InputManager { |
|||
|
|||
public: |
|||
typedef int MenuOption; |
|||
|
|||
private: |
|||
StorageEditor editor; |
|||
|
|||
MenuOption selectedOption; |
|||
|
|||
MenuOption askMenuOption(std::string question, std::vector<std::string> answers); |
|||
|
|||
void displayDialog(std::string question, std::vector<std::string> answers); |
|||
|
|||
struct WareAndAmount { |
|||
Ware ware; |
|||
int amount; |
|||
}; |
|||
|
|||
public: |
|||
|
|||
InputManager(StorageEditor storageEditor); |
|||
|
|||
void displayMainMenu(); |
|||
|
|||
void displayWaren(const std::vector<Ware> waren); |
|||
|
|||
|
|||
WareAndAmount displayAddWareDialog(); |
|||
|
|||
WareAndAmount displayRemoveWareDialog(); |
|||
|
|||
MenuOption getSelectedOption(); |
|||
|
|||
void setSelectedOption(MenuOption option); |
|||
|
|||
template <typename T> |
|||
T getInput(std::string message) const; |
|||
|
|||
bool getNeedsCooling(); |
|||
|
|||
std::vector<WareAndAmount> sortAndGroupWaren(const std::vector<Ware>& waren); |
|||
|
|||
|
|||
|
|||
|
|||
}; |
@ -0,0 +1,26 @@ |
|||
#include "pch.h" |
|||
#include "data-classes/WarenStorage.h" |
|||
|
|||
class StorageEditor { |
|||
private: |
|||
|
|||
WarenStorage storage; |
|||
|
|||
public: |
|||
|
|||
StorageEditor(); |
|||
|
|||
StorageEditor(WarenStorage storage); |
|||
|
|||
WarenStorage getWarenStorage() const; |
|||
|
|||
void setWarenStorage(const WarenStorage storage); |
|||
|
|||
void addWareAndGenerateId(Ware ware); |
|||
|
|||
void addWaren(const Ware ware, int amount); |
|||
|
|||
void removeWare(std::string name); |
|||
|
|||
void removeWaren(std::string name, int amount); |
|||
}; |
@ -0,0 +1,60 @@ |
|||
#include "../pch.h" |
|||
|
|||
class Ware { |
|||
public: |
|||
struct Size { |
|||
double width; |
|||
double height; |
|||
}; |
|||
|
|||
enum WareType { |
|||
DefaultWare, |
|||
CoolingWare, |
|||
LargeWare |
|||
}; |
|||
|
|||
private: |
|||
static int count; |
|||
|
|||
Id id; |
|||
|
|||
bool hasCooling; |
|||
|
|||
std::string name; |
|||
|
|||
Size size; |
|||
|
|||
WareType type; |
|||
|
|||
public: |
|||
Ware(); |
|||
|
|||
~Ware(); |
|||
|
|||
Ware(std::string name, bool hasCooling, double width, double height); |
|||
|
|||
Id getId() const; |
|||
|
|||
void setId(const Id id); |
|||
|
|||
bool getHasCooling() const; |
|||
|
|||
void setHasCooling(const bool isCoolable); |
|||
|
|||
std::string getName() const; |
|||
|
|||
void setName(const std::string name); |
|||
|
|||
Size getSize() const; |
|||
|
|||
void setSize(const double width, const double height); |
|||
|
|||
WareType getType() const; |
|||
|
|||
void setType(const WareType type); |
|||
|
|||
Id generateId(); |
|||
|
|||
bool isLargeWare(Size s); |
|||
|
|||
}; |
@ -0,0 +1,47 @@ |
|||
#include "../pch.h" |
|||
#include "Ware.h" |
|||
|
|||
class WarenStorage { |
|||
public: |
|||
|
|||
//maybe ist das hier einfach garbage |
|||
typedef Ware CoolingWare; |
|||
|
|||
typedef Ware LargeWare; |
|||
|
|||
private: |
|||
|
|||
std::vector<Ware> warenList; |
|||
|
|||
std::vector<CoolingWare> coolingWarenList; |
|||
|
|||
std::vector<LargeWare> largeWarenList; |
|||
|
|||
public: |
|||
|
|||
WarenStorage(); |
|||
|
|||
void addWarenListToSum(std::vector<Ware>* sumList, std::vector<Ware> itemList) const; |
|||
|
|||
std::vector<Ware> getAllWaren() const; |
|||
|
|||
std::vector<Ware> getWarenList(); |
|||
|
|||
void setWarenList(const std::vector<Ware> waren); |
|||
|
|||
std::vector<CoolingWare> getCoolingWarenList(); |
|||
|
|||
void setCoolingWarenList(const std::vector<CoolingWare> coolingWaren); |
|||
|
|||
std::vector<LargeWare> getLargeWarenList(); |
|||
|
|||
void setLargeWarenList(const std::vector<LargeWare> largeWaren); |
|||
|
|||
void addWareAndGenerateId(Ware ware); |
|||
|
|||
void removeWare(const std::string name); |
|||
|
|||
//const frage: |
|||
// |
|||
//wie kann ich die get...Waren() getter wieder als const definieren, ohne dass ich damit StorageEditor die möglichkeit nehme sie zu Editieren. |
|||
}; |
@ -0,0 +1,7 @@ |
|||
#pragma once |
|||
|
|||
#include <iostream> |
|||
#include <string> |
|||
#include <vector> |
|||
|
|||
typedef int Id; |
@ -0,0 +1,14 @@ |
|||
#include "header-files/pch.h"
|
|||
#include "header-files/InputManager.h"
|
|||
|
|||
int Ware::count; |
|||
int main () { |
|||
|
|||
std::cout << "Kaufland:" << std::endl; |
|||
|
|||
StorageEditor editor; |
|||
InputManager inputManager(editor); |
|||
inputManager.displayMainMenu(); |
|||
|
|||
return 0; |
|||
} |
@ -0,0 +1,264 @@ |
|||
#include "../header-files/InputManager.h"
|
|||
#include <exception>
|
|||
#include <sstream>
|
|||
|
|||
InputManager::InputManager(StorageEditor storageEditor) { |
|||
this->editor = storageEditor; |
|||
} |
|||
|
|||
InputManager::MenuOption InputManager::getSelectedOption() { |
|||
return selectedOption; |
|||
} |
|||
|
|||
void InputManager::setSelectedOption(MenuOption option) { |
|||
this->selectedOption = option; |
|||
} |
|||
|
|||
InputManager::MenuOption InputManager::askMenuOption(std::string question, std::vector<std::string> answers) { |
|||
this->displayDialog(question, answers); |
|||
int input; |
|||
std::cin >> input; |
|||
|
|||
return input; |
|||
} |
|||
|
|||
void InputManager::displayDialog(std::string question, std::vector<std::string> answers) { |
|||
std::cout << question << std::endl; |
|||
std::cout << "-------------------------------" << std::endl; |
|||
int counter = 0; |
|||
for(std::string answer:answers) { |
|||
counter++; |
|||
std::cout << counter << ") " << answer << std::endl; |
|||
} |
|||
std::cout << "-------------------------------" << std::endl; |
|||
} |
|||
|
|||
InputManager::WareAndAmount InputManager::displayAddWareDialog() { |
|||
std::string name; |
|||
bool hasCooling; |
|||
double width, height; |
|||
int amount; |
|||
|
|||
name = getInput<std::string>("Enter Ware Name:"); |
|||
hasCooling = getNeedsCooling(); |
|||
width = getInput<double>("Enter Ware width:"); |
|||
height = getInput<double>("Enter Ware height:"); |
|||
amount = getInput<int>("Enter Ware amount:"); |
|||
|
|||
InputManager::WareAndAmount wareAndAmount; |
|||
wareAndAmount.ware = Ware(name, hasCooling, width, height); |
|||
wareAndAmount.amount = amount; |
|||
|
|||
return wareAndAmount; |
|||
} |
|||
|
|||
InputManager::WareAndAmount InputManager::displayRemoveWareDialog() { |
|||
std::string name; |
|||
int amount; |
|||
|
|||
name = getInput<std::string>("Enter Name of the Ware you want to remove:"); |
|||
amount = getInput<int>("Enter amount you want to remove:"); |
|||
|
|||
InputManager::WareAndAmount wareAndAmount; |
|||
|
|||
Ware ware = Ware(); |
|||
ware.setName(name); |
|||
|
|||
wareAndAmount.ware = ware; |
|||
wareAndAmount.amount = amount; |
|||
|
|||
return wareAndAmount; |
|||
} |
|||
|
|||
|
|||
void InputManager::displayMainMenu() { |
|||
std::vector<std::string> answers; |
|||
std::string question = "Wie moechten Sie verfahren?"; |
|||
|
|||
std::string answer = "Ware/n hinzufuegen."; |
|||
answers.push_back(answer); |
|||
|
|||
answer = "Ware/n entfernen."; |
|||
answers.push_back(answer); |
|||
|
|||
answer = "Ware/n anzeigen."; |
|||
answers.push_back(answer); |
|||
|
|||
answer = "Beenden."; |
|||
answers.push_back(answer); |
|||
|
|||
selectedOption = askMenuOption(question, answers); |
|||
switch(selectedOption) { |
|||
case 1: { |
|||
InputManager::WareAndAmount x = displayAddWareDialog(); |
|||
editor.addWaren(x.ware, x.amount); |
|||
break; |
|||
} |
|||
case 2: { |
|||
InputManager::WareAndAmount x = displayRemoveWareDialog(); |
|||
editor.removeWaren(x.ware.getName(), x.amount); |
|||
break; |
|||
} |
|||
case 3: { |
|||
std::vector<Ware> waren = this->editor.getWarenStorage().getAllWaren(); |
|||
displayWaren(waren); |
|||
break; |
|||
} |
|||
case 4: { |
|||
return; |
|||
break; |
|||
} |
|||
} |
|||
|
|||
displayMainMenu(); |
|||
} |
|||
|
|||
// if no more initialization needed --> refactor to setStorageEditor
|
|||
void InputManager::displayWaren(const std::vector<Ware> waren) { |
|||
std::cout << "Liste der sich im Lager befindlichen Waren:" << std::endl; |
|||
std::vector<InputManager::WareAndAmount> sortedWarenList = sortAndGroupWaren(waren); |
|||
|
|||
if(sortedWarenList.empty()){ |
|||
std::cout << "Das Warenlager ist leer." << std::endl; |
|||
std::cout << "---------------------" << std::endl; |
|||
return; |
|||
} |
|||
|
|||
for(WareAndAmount wareAndAmount: sortedWarenList){ |
|||
std::cout << "Warenname:" << std::endl; |
|||
std::cout << wareAndAmount.ware.getName() << std::endl; |
|||
|
|||
std::cout << "Needs Cooling:" << std::endl; |
|||
if(wareAndAmount.ware.getHasCooling()) { |
|||
std::cout << "true" << std::endl; |
|||
} |
|||
else { |
|||
std::cout << "false" << std::endl; |
|||
} |
|||
|
|||
std::cout << "Width:" << std::endl; |
|||
std::cout << wareAndAmount.ware.getSize().width << std::endl; |
|||
|
|||
std::cout << "Height:" << std::endl; |
|||
std::cout << wareAndAmount.ware.getSize().height << std::endl; |
|||
|
|||
std::cout << "Amount:" << std::endl; |
|||
std::cout << wareAndAmount.amount << std::endl; |
|||
|
|||
std::cout << "---------------------" << std::endl; |
|||
} |
|||
} |
|||
|
|||
//refactor
|
|||
std::vector<InputManager::WareAndAmount> InputManager::sortAndGroupWaren(const std::vector<Ware>& waren) { |
|||
std::vector<WareAndAmount> sortedWaren; |
|||
|
|||
WareAndAmount wareAndAmount; |
|||
wareAndAmount.amount = 0; |
|||
|
|||
for(Ware ware : waren) { |
|||
if(ware.getType() == Ware::WareType::DefaultWare) { |
|||
if(wareAndAmount.amount == 0) { |
|||
wareAndAmount.ware = ware; |
|||
wareAndAmount.amount++; |
|||
} |
|||
else if(wareAndAmount.ware.getName() == ware.getName()) { |
|||
wareAndAmount.amount++; |
|||
} |
|||
else { |
|||
sortedWaren.push_back(wareAndAmount); |
|||
wareAndAmount.amount = 0; |
|||
} |
|||
} |
|||
} |
|||
if(!waren.empty() && wareAndAmount.amount > 0){ |
|||
sortedWaren.push_back(wareAndAmount); |
|||
wareAndAmount.amount = 0; |
|||
} |
|||
|
|||
for(Ware ware : waren) { |
|||
if(ware.getType() == Ware::WareType::CoolingWare) { |
|||
if(wareAndAmount.amount == 0) { |
|||
wareAndAmount.ware = ware; |
|||
wareAndAmount.amount++; |
|||
} |
|||
else if(wareAndAmount.ware.getName() == ware.getName()) { |
|||
wareAndAmount.amount++; |
|||
} |
|||
else { |
|||
sortedWaren.push_back(wareAndAmount); |
|||
wareAndAmount.amount = 0; |
|||
} |
|||
} |
|||
} |
|||
if(!waren.empty() && wareAndAmount.amount > 0){ |
|||
sortedWaren.push_back(wareAndAmount); |
|||
wareAndAmount.amount = 0; |
|||
} |
|||
|
|||
for(Ware ware : waren) { |
|||
if(ware.getType() == Ware::WareType::LargeWare) { |
|||
if(wareAndAmount.amount == 0) { |
|||
wareAndAmount.ware = ware; |
|||
wareAndAmount.amount++; |
|||
} |
|||
else if(wareAndAmount.ware.getName() == ware.getName()) { |
|||
wareAndAmount.amount++; |
|||
} |
|||
else { |
|||
sortedWaren.push_back(wareAndAmount); |
|||
wareAndAmount.amount = 0; |
|||
} |
|||
} |
|||
} |
|||
if(!waren.empty() && wareAndAmount.amount > 0){ |
|||
sortedWaren.push_back(wareAndAmount); |
|||
wareAndAmount.amount = 0; |
|||
} |
|||
|
|||
return sortedWaren; |
|||
} |
|||
|
|||
|
|||
bool InputManager::getNeedsCooling() { |
|||
std::string input; |
|||
bool hasCooling; |
|||
|
|||
std::cout << "Does Ware need Cooling? (y/n)" << std::endl; |
|||
try { |
|||
std::cin >> input; |
|||
if(input == "y") { |
|||
hasCooling = true; |
|||
} |
|||
else if(input == "n") { |
|||
hasCooling = false; |
|||
} |
|||
else { |
|||
std::cout << "That didn't work. Please try again :)" << std::endl; |
|||
getNeedsCooling(); |
|||
} |
|||
} |
|||
catch(const std::exception&){ |
|||
std::cout << "That didn't work. Please try again :)" << std::endl; |
|||
getNeedsCooling(); |
|||
} |
|||
return hasCooling; |
|||
} |
|||
|
|||
//std::istream
|
|||
template <typename T> |
|||
T InputManager::getInput(std::string message) const { |
|||
std::cout << message << std::endl; |
|||
T val; |
|||
try { |
|||
std::string input; |
|||
std::cin >> input; |
|||
std::istringstream ss(input); |
|||
ss >> val; |
|||
} |
|||
catch(const std::exception&) { |
|||
std::cout << "That didn't work. Please try again :)" << std::endl; |
|||
getInput<T>(message); |
|||
} |
|||
return val; |
|||
} |
@ -0,0 +1,30 @@ |
|||
#include "../header-files/StorageEditor.h"
|
|||
|
|||
StorageEditor::StorageEditor() { } |
|||
|
|||
StorageEditor::StorageEditor(const WarenStorage storage) { |
|||
this->storage = storage; |
|||
} |
|||
|
|||
WarenStorage StorageEditor::getWarenStorage() const { |
|||
return storage; |
|||
} |
|||
|
|||
void StorageEditor::setWarenStorage(const WarenStorage storage) { |
|||
this->storage = storage; |
|||
} |
|||
|
|||
|
|||
void StorageEditor::addWaren(const Ware ware, int amount) { |
|||
for(auto i = 0; i < amount; i++) { |
|||
storage.addWareAndGenerateId(ware); |
|||
} |
|||
} |
|||
|
|||
|
|||
void StorageEditor::removeWaren(std::string name, const int amount) { |
|||
for(auto i = 0; i < amount; i++) { |
|||
storage.removeWare(name); |
|||
} |
|||
} |
|||
|
@ -0,0 +1,69 @@ |
|||
#include "../header-files/data-classes/Ware.h"
|
|||
|
|||
Ware::Ware(){ ++count; } |
|||
Ware::~Ware() { --count; } |
|||
|
|||
Ware::Ware(std::string name, bool hasCooling, double width, double height) { |
|||
this->name = name; |
|||
this->hasCooling = hasCooling; |
|||
this->size = { width, height }; |
|||
|
|||
if(hasCooling) { |
|||
this->type = CoolingWare; |
|||
} else if(isLargeWare(this->size)) { |
|||
this->type = LargeWare; |
|||
} else { |
|||
this->type = DefaultWare; |
|||
} |
|||
count++; |
|||
this->id = this->generateId(); |
|||
} |
|||
|
|||
Id Ware::getId() const { |
|||
return id; |
|||
} |
|||
|
|||
void Ware::setId(const Id id) { |
|||
this->id = id; |
|||
} |
|||
|
|||
Ware::WareType Ware::getType() const { |
|||
return type; |
|||
} |
|||
|
|||
void Ware::setType(const WareType type) { |
|||
this->type = type; |
|||
} |
|||
|
|||
bool Ware::getHasCooling() const { |
|||
return hasCooling; |
|||
} |
|||
|
|||
void Ware::setHasCooling(const bool hasCooling) { |
|||
this->hasCooling = hasCooling; |
|||
} |
|||
|
|||
std::string Ware::getName() const { |
|||
return name; |
|||
} |
|||
|
|||
void Ware::setName(const std::string name) { |
|||
this->name = name; |
|||
} |
|||
|
|||
Ware::Size Ware::getSize() const { |
|||
return size; |
|||
} |
|||
|
|||
void Ware::setSize(const double width, const double height) { |
|||
this->size = Size{ width, height }; |
|||
} |
|||
|
|||
bool Ware::isLargeWare(Size s) { |
|||
return (s.width > 20.0 || s.height > 20.0); |
|||
} |
|||
|
|||
Id Ware::generateId() { |
|||
//billige id generation da keine reference benutzt
|
|||
return (uint64_t) &this->name; |
|||
} |
@ -0,0 +1,92 @@ |
|||
#include "../header-files/data-classes/WarenStorage.h"
|
|||
|
|||
|
|||
WarenStorage::WarenStorage() { } |
|||
|
|||
void WarenStorage::addWarenListToSum(std::vector<Ware>* sumList, std::vector<Ware> itemList) const { |
|||
//reference ohne const möglich oder ist hier ein pointer notwendig??
|
|||
for(Ware ware : itemList) { |
|||
sumList->push_back((Ware) ware); //static_cast<> oder ganz weg
|
|||
} |
|||
} |
|||
|
|||
std::vector<Ware> WarenStorage::getAllWaren() const { |
|||
|
|||
std::vector<Ware> w; |
|||
|
|||
addWarenListToSum(&w, warenList); |
|||
addWarenListToSum(&w, coolingWarenList); |
|||
addWarenListToSum(&w, largeWarenList); |
|||
|
|||
return w; |
|||
} |
|||
|
|||
std::vector<Ware> WarenStorage::getWarenList() { |
|||
return warenList; |
|||
} |
|||
|
|||
void WarenStorage::setWarenList(const std::vector<Ware> waren) { |
|||
this->warenList = waren; |
|||
} |
|||
|
|||
std::vector<WarenStorage::CoolingWare> WarenStorage::getCoolingWarenList() { |
|||
return coolingWarenList; |
|||
} |
|||
|
|||
void WarenStorage::setCoolingWarenList(const std::vector<CoolingWare> coolingWaren) { |
|||
this->coolingWarenList = coolingWaren; |
|||
} |
|||
|
|||
std::vector<Ware> WarenStorage::getLargeWarenList() { |
|||
return largeWarenList; |
|||
} |
|||
|
|||
void WarenStorage::setLargeWarenList(const std::vector<LargeWare> largeWaren) { |
|||
this->largeWarenList = largeWaren; |
|||
} |
|||
|
|||
void WarenStorage::addWareAndGenerateId(Ware ware) { |
|||
|
|||
ware.generateId(); |
|||
switch(ware.getType()) { |
|||
case Ware::WareType::DefaultWare: |
|||
warenList.push_back(ware); |
|||
break; |
|||
|
|||
case Ware::WareType::CoolingWare: |
|||
coolingWarenList.push_back(ware); |
|||
break; |
|||
|
|||
case Ware::WareType::LargeWare: |
|||
largeWarenList.push_back(ware); |
|||
break; |
|||
} |
|||
} |
|||
|
|||
void WarenStorage::removeWare(const std::string name) { |
|||
std::vector<Ware> allWaren = getAllWaren(); |
|||
for(size_t i = 0; i < allWaren.size(); i++) { |
|||
if(allWaren[i].getName() == name) { |
|||
switch(allWaren[i].getType()) { |
|||
case Ware::WareType::DefaultWare: |
|||
warenList.erase( |
|||
warenList.begin() + i |
|||
); |
|||
break; |
|||
|
|||
case Ware::WareType::CoolingWare: |
|||
coolingWarenList.erase( |
|||
coolingWarenList.begin() + i |
|||
); |
|||
break; |
|||
|
|||
case Ware::WareType::LargeWare: |
|||
largeWarenList.erase( |
|||
largeWarenList.begin() + i |
|||
); |
|||
break; |
|||
} |
|||
break; //remove only one item
|
|||
} |
|||
} |
|||
} |
Write
Preview
Loading…
Cancel
Save
Reference in new issue